C# is a powerful and versatile language, widely used for developing a range of applications, from desktop to web and mobile. However, even seasoned developers can fall into common pitfalls that lead to bugs, performance issues, and maintainability challenges.

In this blog post, we will explore some of the most frequent mistakes made in C# programming, understand why they occur, and learn practical strategies to avoid and fix them. Whether you’re a beginner or an experienced developer, this guide will help you write cleaner, more efficient, and reliable C# code.
Here are code examples demonstrating the mistakes and their fixes:
#1 Null Reference Exceptions
⚠️Wrong:

✔️Right:

#2 Ignoring Exceptions
⚠️Wrong:

✔️Right:

#3 String Concatenation in Loops
⚠️Wrong:

✔️Right:

#4 Not Disposing IDisposable Objects
⚠️Wrong:

✔️Right:

#5 Inefficient LINQ Queries
⚠️Wrong:

✔️Right:

#6 Incorrect Use of Async/Await
⚠️Wrong:

✔️Right:

#7 Mutable Structs
⚠️Wrong:

✔️Right:

#8 Directly Modifying foreach Loop Variables
⚠️Wrong:

✔️Right:

#9 Incorrectly Handling Timezones
⚠️Wrong:

✔️Right:

#10 Ignoring Code Analysis Warnings
⚠️Wrong:

✔️Right:

#11 Not Using Dependency Injection
⚠️Wrong:

✔️Right:

#12 Overusing Singletons
⚠️Wrong:
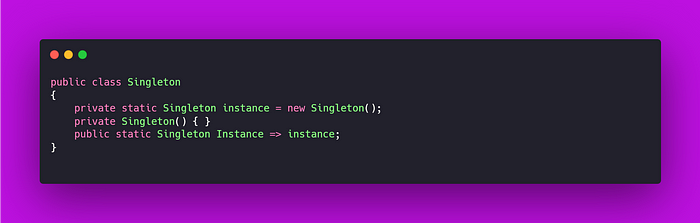
✔️Right:

#13 Ignoring Localization
⚠️Wrong:

✔️Right:

#14 Inefficient Data Access
⚠️Wrong:

✔️Right:
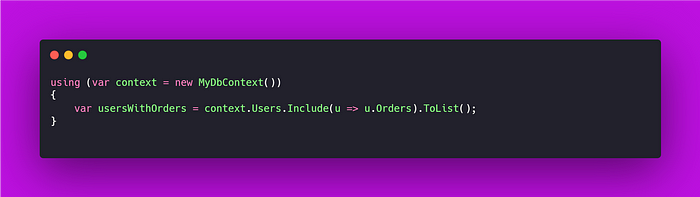
#15 Excessive Logging
⚠️Wrong:

✔️Right:

#16 Ignoring Security Practices
⚠️Wrong:

✔️Right:

#17 Hardcoding Configuration Settings
⚠️Wrong:

✔️Right:
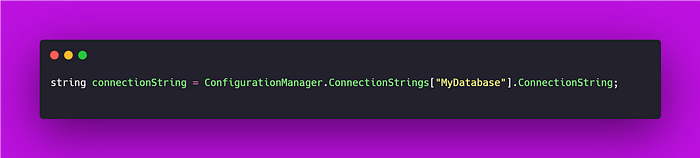
#18 Lack of Unit Tests
⚠️Wrong:
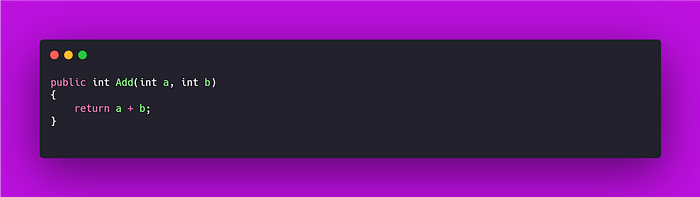
✔️Right:

#19 Mixing Business Logic with Presentation Logic
⚠️Wrong:
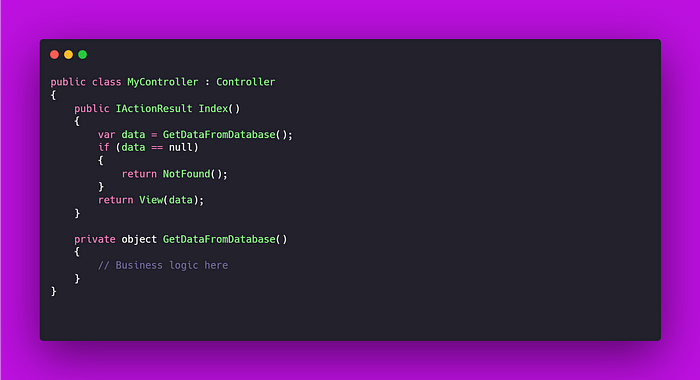
✔️Right:

#20 Ignoring Memory Management
⚠️Wrong:

✔️Right:

#21 Not Following Coding Standards
⚠️Wrong:

✔️Right:

Avoiding common programming mistakes in C# is crucial for developing robust, efficient, and maintainable applications. By understanding these pitfalls and implementing best practices, you can significantly reduce bugs and enhance your code quality. Remember, continual learning and code review are key to improving your skills. Keep refining your techniques, and don’t hesitate to leverage tools and resources that can help you identify and fix errors early. With diligence and attention to detail, you can become a more proficient C# developer and create applications that stand the test of time.